INSIGHTS
Mastering Web Development with the Intersection Observer API
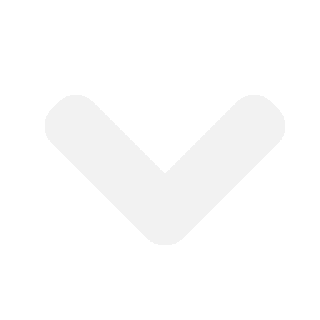
By: Robert Kegel
Creating dynamic and interactive web experiences is at the core of modern web development. The Intersection Observer API is a powerful tool that enables developers to efficiently track and respond to changes in the visibility of DOM elements. In this comprehensive guide, we will delve into the intricacies of the Intersection Observer API, providing a deeper understanding of its key concepts and demonstrating practical examples of how it can be harnessed to enhance a navigation menu by activating corresponding items when their linked sections become visible during scroll.
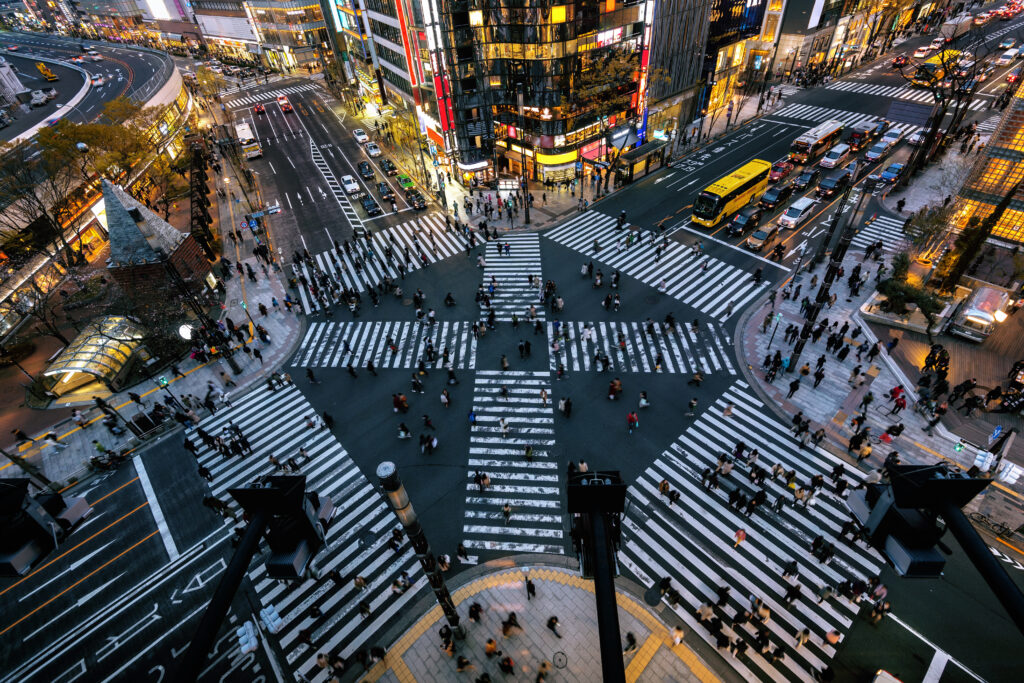
Table of Contents:
- Understanding the Intersection Observer API
- Key Concepts
- Basic Usage
- Observing Elements and Intersection Ratios
- Using the Intersection Observer API for Navigation Menus
- Conclusion
Understanding the Intersection Observer API:
The Intersection Observer API offers a straightforward and efficient method for asynchronously observing changes in the intersection between a target element and a parent container or viewport. It simplifies the process of detecting when an element enters or exits a specified boundary, making it an invaluable tool for building responsive and interactive web applications.
Traditionally, developers relied on scroll event listeners or polling techniques to determine element visibility. However, these methods often resulted in performance issues, especially when monitoring a large number of elements. The Intersection Observer API addresses these challenges by offloading the responsibility of tracking visibility changes to the browser, ensuring optimal performance and accuracy.
Key Concepts:
Before diving into the practical implementation, let’s explore some key concepts associated with the Intersection Observer API:
Target Element: The DOM element being observed for visibility changes.
Intersection Observer: An instance of the `IntersectionObserver` class that tracks the intersection between the target element and the specified boundary.
Intersection Observer Entry: An object of the `IntersectionObserverEntry` class that encapsulates information about a target element’s visibility state, such as the intersection ratio, bounding rectangle, and more.
Threshold: A value between 0 and 1 that determines the proportion of the target element that must be visible within the boundary to trigger the intersection callback.
Intersection Ratio: The ratio of the visible portion of the target element relative to its total size. It ranges from 0 (element fully outside the boundary) to 1 (element fully within the boundary).
Callback Function: A function that is executed whenever an observed element intersects or stops intersecting with the specified boundary. It receives an array of `IntersectionObserverEntry` objects as its parameter.
Root Margin: An additional margin applied to the root element’s bounding box, expanding or contracting the intersection area. It allows for more flexible control over when the intersection callback is triggered.
Basic Usage:
To begin utilizing the Intersection Observer API, we need to create an instance of the `IntersectionObserver` class, passing in a callback function and optional configuration options.
const observer = new IntersectionObserver(callback, options);
The `callback` function is called whenever an observed element intersects or stops intersecting with the specified boundary. It receives an array of `IntersectionObserverEntry` objects, allowing us to access information about each observed element.
const callback = (entries) => {
entries.forEach((entry) => {
// Perform actions based on entry data
});
};
Observing Elements and Intersection Ratios:
To observe a specific element, we use the `observe()` method of the `IntersectionObserver` instance, passing in the target element as an argument.
const targetElement = document.getElementById(‘target’);
observer.observe(targetElement);
Once the observed element intersects or exits the specified boundary, the callback function specified during the IntersectionObserver instance creation is triggered. Within the callback function, we can access an array of IntersectionObserverEntry objects through the entries parameter.
const callback = (entries) => {
entries.forEach((entry) => {
// Perform actions based on entry data
});
};
Now, let’s explore the intersectionRatio property provided by the IntersectionObserverEntry object. The intersectionRatio represents the ratio of the visible portion of the observed element relative to its total size. It ranges from 0 (element fully outside the boundary) to 1 (element fully within the boundary).
By utilizing the intersectionRatio, we can create dynamic behaviors based on how much of an element is visible. For example, we can fade in an element when it becomes partially visible or trigger an animation when it becomes fully visible.
Here’s an example that demonstrates the usage of the intersectionRatio property:
<div class="fade-in-element">
<!-- Content -->
</div>
.fade-in-element {
opacity: 0;
transition: opacity 0.5s ease;
}
.fade-in-element.active {
opacity: 1;
}
const element = document.querySelector('.fade-in-element');
const options = {
threshold: 0.5, // Set the threshold as needed
};
const callback = (entries) => {
entries.forEach((entry) => {
if (entry.isIntersecting && entry.intersectionRatio >= 0.5) {
entry.target.classList.add('active');
} else {
entry.target.classList.remove('active');
}
});
};
const observer = new IntersectionObserver(callback, options);
observer.observe(element);
In the above example, we have a div element with the class fade-in-element that initially has an opacity of 0. We want to fade it in when it becomes at least 50% visible.
We create an instance of the IntersectionObserver with the callback function and specify a threshold of 0.5, indicating that the callback should be triggered when at least 50% of the element is visible.
Within the callback function, we check if the observed element is intersecting the boundary (entry.isIntersecting) and if the intersectionRatio is greater than or equal to 0.5. If both conditions are met, we add the active class to the element, which applies the CSS transition and gradually increases its opacity.
By adjusting the threshold and utilizing the intersectionRatio, we can fine-tune the timing and behavior of various interactive elements based on their visibility. Remember to experiment and adapt the intersectionRatio threshold and corresponding actions to achieve the desired effects in your web applications.
Continue to the next section for an exciting practical application of the Intersection Observer API in navigation menus.
Using the Intersection Observer API for Navigation Menus:
A practical application of the Intersection Observer API is creating an active navigation menu that highlights the currently visible section. Let’s explore an example:
<nav id="navMenu">
<ul>
<li><a href="#section1">Section 1</a></li>
<li><a href="#section2">Section 2</a></li>
<li><a href="#section3">Section 3</a></li>
</ul>
</nav>
<section id="section1">
<!-- Content for Section 1 -->
</section>
<section id="section2">
<!-- Content for Section 2 -->
</section>
<section id="section3">
<!-- Content for Section 3 -->
</section>
const navItems = document.querySelectorAll('#navMenu li a');
const sections = document.querySelectorAll('section');
const options = {
threshold: 0.5, // Adjust this value based on your requirements
rootMargin: '-50px' // Adjust this value based on your layout and design
};
const callback = (entries) => {
entries.forEach((entry) => {
if (entry.isIntersecting) {
const targetId = entry.target.getAttribute('id');
activateNavItem(targetId);
}
});
};
const observer = new IntersectionObserver(callback, options);
sections.forEach((section) => {
observer.observe(section);
});
function activateNavItem(targetId) {
navItems.forEach((item) => {
item.classList.toggle('active', item.getAttribute('href') === `#${targetId}`);
});
}
For a similar example with interactive code, you can check out this CodePen. It demonstrates the usage of the Intersection Observer API to create an active navigation menu that highlights the currently visible section.
In the above example, we start by selecting all the navigation menu items and sections using appropriate CSS selectors. Next, we create an instance of the `IntersectionObserver` with the `callback` function and configuration options.
The `options` object includes the `threshold` property, which determines the proportion of the section that must be visible within the viewport to trigger the intersection callback. You can adjust this value based on your requirements.
Additionally, we introduce the `rootMargin` property, which allows you to define an extra margin around the root element’s bounding box. This margin expands or contracts the intersection area, providing more control over when the intersection callback is triggered. Adjust the value of `rootMargin` according to your layout and design needs.
We then loop through each section and observe them using the `observer.observe()` method. When a section intersects the boundary, the `callback` function is triggered. Within the callback, we check if the observed section is intersecting (`entry.isIntersecting`), and if so, we retrieve its ID and call the `activateNavItem()` function.
The `activateNavItem()` function iterates over the navigation menu items and toggles the ‘active’ class based on whether the item’s `href` attribute matches the target section’s ID.
By dynamically updating the ‘active’ class based on section visibility, we create an interactive navigation menu that highlights the active section during scrolling.
Conclusion:
The Intersection Observer API is a powerful tool that empowers web developers to create engaging and responsive user experiences. By leveraging this API, we can efficiently track the visibility of DOM elements and build interactive websites that adapt to user actions.
In this post, we explored the Intersection Observer API’s fundamental concepts, basic usage, and demonstrated a practical example of utilizing it to create an active navigation menu. By applying this knowledge, you can elevate your web development skills and deliver exceptional user experiences.
Remember to experiment and customize the API’s options, such as the `threshold` and `rootMargin`, to suit your specific requirements and achieve the desired behavior.